ERC-20 Tokens
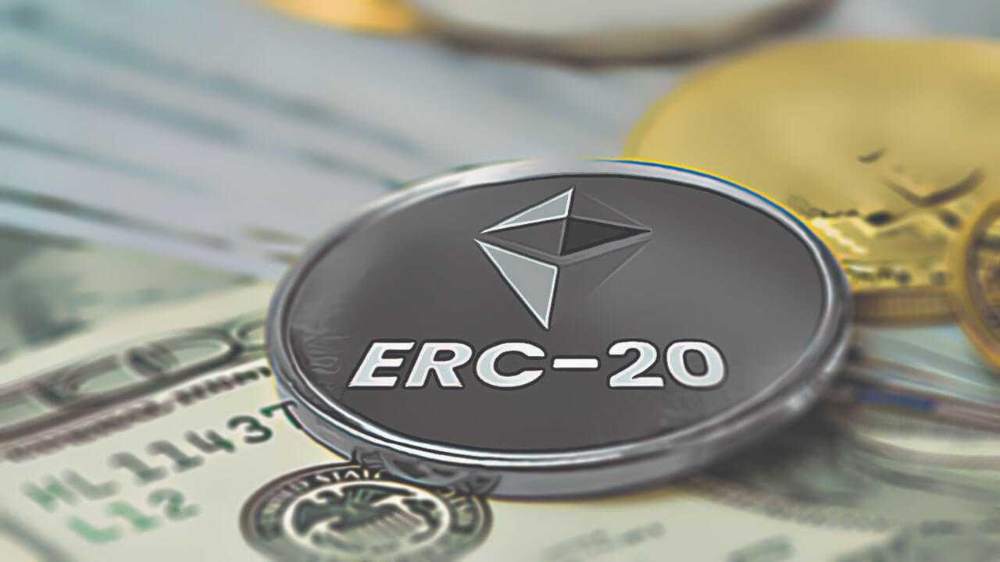
What is the ERC-20 standard?
In Ethereum, ERC stands for Ethereum Requests for Comments . These are technical documents that outline standards for programming on Ethereum. ERC should not be confused with Ethereum Improvement Proposals (EIPs), which propose improvements to the protocol itself, like Bitcoin's BIPs. ERC aims to create order to allow applications and contracts to interact with each other more easily.
Written by Vitalik Buterin and Fabian Vogelsteller in 2015, ERC-20 introduces a relatively simpler format for Ethereum-based tokens. When developers follow this framework, they don't have to start all over again. Instead, they can build on a foundation already used throughout the industry.
When new ERC-20 tokens are created, they automatically become interoperable with software and services that support the ERC-20 standard ( software wallets , hardware wallets , exchanges , etc.).
It should be noted that the ERC-20 standard is built on an EIP (EIP-20 to be exact). This development occurred due to widespread use, several years after the original proposal. But even years later, the name "ERC-20" is still used.
A brief summary of Ethereum tokens
Unlike ETH (Ethereum's native currency), ERC-20 tokens are not held by accounts. Tokens exist within only one contract, which is similar to a self-contained database. It sets the rules for tokens (e.g. name, symbol, divisibility) and maintains a list linking users' balances to their Ethereum addresses.
To move tokens, users must submit a transaction to the contract requesting that some of their balance be transferred elsewhere. For example, if Alice wants to send 5,000 BinanceAcademyTokens to Bob, she requests this transaction by calling a function within the BinanceAcademyToken smart contract.
The call will be inside a transaction that appears to be a standard Ethereum transaction paying 0 ETH into the token contract. This call is included in an additional part of the transaction and specifies what Alice wants to do – in our example, Alice's request is to transfer the tokens to Bob.
Even though he is not sending Ether, he still has to pay a fee in ETH for his transaction to be included in a block . If he doesn't have ETH, he needs to buy some ether before transferring the tokens.
Here is a real-life example of what we talked about above on Etherscan : A person places a call on the BUSD contract. You can see that tokens have been transferred and a transaction fee has been paid, whereas the Value field shows that 0 ETH has been sent.
Now that we've gone over some of the basics, we can go into a little more detail to understand the structure of a typical ERC-20 contract.
How are ERC-20 tokens created?
To be ERC-20 compliant, the contract must include six mandatory functions: totalSupply (total supply), balanceOf (balance), transfer (transfer), transferFrom (source of transfer), approve (approval) and allowance (remaining balance). In addition, you can specify optional functions such as name , symbol and decimal . You can understand what these functions do from their names. It's okay if you don't understand, we will examine each one in detail.
The functions as they appear in Ethereum's purpose-built Solidity language consist of:
totalSupply
function totalSupply() public view returns (uint256)
When the above function is called by a user, it returns the total supply of tokens on the contract .
balanceOf
function balanceOf(address _owner) public view returns (uint256 balance)
Unlike totalSupply , balanceOf takes one parameter (an address). When called, it returns the balance of this address's token holdings. Remember that accounts on the Ethereum network are public, so you can query the balance of any user as long as you know their address.
transfer
function transfer(address _to, uint256 _value) public returns (bool success)
Transfer transfers tokens from one user to another in a convenient manner. Here you need to specify the address you want to ship to and the amount to be sent.
When the transfer is called, it triggers an event (in this case, the transfer event) that tells the blockchain to add a reference given to it.
transferFrom
function transferFrom(address _from, address _to, uint256 _value) public returns (bool success)
The transferFrom functionis a useful alternative to transfer that offers a little more programmability in decentralized applications. Just like the transfer, this function is used to move tokens, but these tokens do not need to belong to the person calling the contract.
In other words, you can authorize a person – or another contract – to transfer funds on your behalf. One possible use case is subscription-based services where you don't want to manually make payments every day/week/month. Instead of making the payment yourself, you let the program do it for you.
This function triggers the same event as transfer .
approve
function approve(address _spender, uint256 _value) public returns (bool success)
approve is another useful function for programmability. With this function, you can limit the number of tokens that a smart contract can withdraw from your balance. Without this function, you run the risk of the contract malfunctioning (or being hijacked) and stealing all your funds.
Let's go back to our subscription model example. Let's say you have a very large amount of BinanceAcademyTokens and want to make weekly recurring payments to a streaming DApp . Since you are busy reading Binance Academy content day and night, you do not want to deal with making a manual transaction every week.
Your BinanceAcademyTokens balance is too high, much more than you need to pay for the subscription. To prevent the DApp from taking all your tokens, you can set a limit using the approve function. Let's say you pay one BinanceAcademyToken weekly towards your subscription. If you set the upper limit of the approved amount at twenty tokens, you can have your subscription automatically paid for five months.
In the worst case scenario, if the DApp tries to withdraw all your funds or a software vulnerability is found, you will only lose twenty tokens. Of course, this situation won't be ideal, but it's much better than losing all your savings.
When approve is called, it triggers an approval event. This event, like the transfer event, writes data onto the blockchain.
allowance
function allowance(address _owner, address _spender) public view returns (uint256 remaining)
allowance can be used with(remaining balance) approve . When you allow a contract to manage your tokens , you may want to check how many tokens the contract has left to withdraw. For example, if your subscription used twelve of your twenty confirmed tokens,you would get eight in response when you call the allowance function.
Optional functions
The functions we mentioned above are mandatory. On the other hand, it is not necessary to include name , symbol , and decimal , but these features can make your ERC-20 contract a little better. These functions respectively allow you to add a human-readable name, specify a symbol (e.g. ETH, BTC, BNB), and specify how many decimal units the token can be divided into. For example, greater divisibility is more important for tokens used as currency than for a token that represents ownership.
To examine these elements on a real contract, you can check out this example on GitHub.
What can ERC-20 tokens do?
When we put all the above functions together, we have an ERC-20 contract. We can query the total supply , check balances, transfer funds and allow other Dapps to manage our tokens on our behalf.
The appeal of ERC-20 tokens is largely based on their flexibility. The current setup does not limit the ability to make improvements, so parties can implement additional features and set specific parameters based on their needs.
stablecoins
Stablecoins (tokens whose value is pegged to fiat currencies ) often use the ERC-20 token standard. The transaction to the BUSD contract we mentioned earlier is an example of this, and most major stablecoins also use this format.
In a typical fiat-backed stablecoin, the issuer of the coin is worth the euro, dollar, etc. It has reserves. It then issues one token for each unit in its reserve. So, if 10,000 USD is locked in a vault, the issuing institution can create 10,000 tokens that can be exchanged for 1USD each.
Technically speaking, this is pretty easy to implement in Ethereum. It is sufficient for the issuing institution to issue a contract with 10,000 tokens. These tokens are then distributed to users with the promise that they can be converted into fiat currencies at a one-to-one ratio in the future.
Users can do many things with their tokens – buy products and services or use the tokens in Dapps. Instead, they can also ask the issuing institution to convert the tokens immediately. At this point, the issuing institution burns the returned tokens (rendering them unusable) and withdraws the corresponding amount of fiat from its reserve.
The contract that governs this system is quite simple, as we mentioned before. But launching a stablecoin requires a lot of work on logistics, regulatory compliance, and many other external factors.
security tokens
Security tokens are similar to stablecoins. Since they both work the same way, they can be exactly the same at the contract level. The difference occurs at the extractive institution level. Security tokens represent securities such as stocks, bonds, and physical assets. Often (but not always), they allocate a stake in a company or product to the token holder.
service tokens
Utility tokens are probably the most common type of tokens today. Unlike the other two tokens, utility tokens are not backed by anything. If we compare asset-backed tokens to shares in an airline, then service tokens would be similar to frequent flyer programs: they serve a purpose, but have no external value. Utility tokens have many uses and can serve many purposes such as in-game currency , means of payment for decentralized applications, loyalty points, and more.
Can ERC-20 tokens be mined?
You can mine Ether (ETH), but the tokens are not minable – the creation of new tokens is called minting. Once a contract is created, developers distribute the supply according to their plans and roadmaps.
This is typically done through an Initial Coin Offering (ICO), Initial Exchange Offering (IEO) or Security Token Offering (STO). You may encounter various versions of these acronyms, but the concepts will be quite similar. Investors send ether to the contract address and receive new tokens in return. The money collected is spent on the development of the project. Users expect to be able to redeem their tokens (either immediately or at a later date) or sell their tokens to make a profit as the project develops.
Token distribution does not need to be automatic. Many fundraising events allow users to pay with different digital currencies (such as BNB, BTC, ETH and USDT). Then, the corresponding amount of balance is transferred to the addresses provided by the users.
Advantages and disadvantages of ERC-20 tokens
Advantages of ERC-20 tokens
can be exchanged
ERC-20 tokens are fugible – all units are interchangeable. If you own a BinanceAcademyToken, it doesn't matter which specific token you own. You can swap your token for another person's token and, similar to cash or gold, your token will work the same way as before.
This is ideal if your tokens aim to become a form of currency. You don't want units to have properties that differ from each other, because then they become non-exchangeable. If there is variation, some tokens may become more or less valuable than others, damaging the underlying purpose.
Flexibility
As we explored in the previous section, ERC-20 tokens can be highly customizable and compatible with many different applications. For example, they can be used as in-game currency, in loyalty point programs, as digital collectibles , and even to represent works of art and property rights.
Popularity
The popularity of ERC-20 in the cryptocurrency industry is a very compelling reason to use this standard as a blueprint. There will be a multitude of exchanges, wallets and smart contracts already compatible with the newly issued tokens. What's more, developer support and documentation is easy to access.
Disadvantages of ERC-20 tokens
Scalability
Like many cryptocurrency networks, Ethereum has issues with growth. In its current form, it is not very scalable – trying to send a transaction during peak times can result in high transaction fees and delays. If you issue an ERC-20 token and the network becomes congested, the usability of the token will be affected.
This problem is not unique to Ethereum. In fact, scalability is a necessary balance of profit and loss for secure and distributed systems. The community plans to move to Ethereum 2.0, which will implement upgrades such as Ethereum Plasma and Ethereum Casper to address these issues.
You can read more about scalability issues in our article Blockchain Scalability: Sidechains and Payment Channels .
Scams
Although this is not a problem with the technology itself, the ease of issuing a token can be a disadvantage in some ways. It is possible to issue a simple ERC-20 token with minimal effort, meaning anyone can do it in good faith or bad faith.
So you need to be careful about what you invest in. There are many Pyramid and Ponzi schemes hidden under the name of Blockchain projects . It is very important that you do your own research before investing and make your own conclusion as to whether the opportunity before you is legitimate.
The ERC-20 standard has been dominant in the cryptoasset world for years, and it's easy to see why. Anyone can pretty easily create a simple contract suitable for many different use cases (utility token, stablecoin, etc.). However, ERC-20 does not have some features offered by other standards. Only time can tell whether later contract types will replace ERC-20.
https://www.bulbapp.io/u/BYqeEQZZWzQn7MVpopges4zH3qe3yeNDunPVY9f3FixS/erendurden